Chapter 13 Message boxes
13.1 Message box parameters
We have seen that much of the format of a message box can be specified by a single numerical parameter. Here we will review the possible values which can make up this parameter, and illustrate them with a few examples.
First we consider the most basic requirement: the number and type of buttons which should appear. The possible combinations and associated values are listed below.
Constant | Value | Description |
---|---|---|
vbOKOnly |
0 | OK button only |
vbOKCancel |
1 | OK and Cancel buttons |
vbAbortRetryIgnore |
2 | Abort, Retry, and Ignore buttons |
vbYesNoCancel |
3 | Yes, No, and Cancel buttons |
vbYesNo |
4 | Yes and No buttons |
vbRetryCancel |
5 | Retry and Cancel buttons |
Next we consider the possible icons which can precede the prompt.
Constant | Value | Description |
---|---|---|
vbCritical |
16 | Display Critical Message icon |
vbQuestion |
32 | Display Warning Query icon |
vbExclamation |
48 | Display Warning Message icon |
vbInformation |
64 | Display Info Message icon |
We can also set which button will be the default response
(corresponding to pressing Return
).
Constant | Value | Description |
---|---|---|
vbDefaultButton1 |
0 | First button is default |
vbDefaultButton2 |
256 | Second button is default |
vbDefaultButton3 |
512 | Third button is default |
Finally we can control the behaviour of the message box.
Constant | Value | Description |
---|---|---|
vbApplicationModal |
0 | The application stops until the user responds |
vbSystemModal |
4096 | The whole system stops until the user responds |
vbMsgBoxHelpButton |
16384 | A Help button is added to the message box |
vbMsgBoxSetForeground |
65536 | The Message Box is put in the foreground |
vbMsgBoxRight |
524288 | The text in the Message Box is right aligned |
vbMsgBoxRtlReading |
1048576 | The text is presented right to left (for Arabic and Hebrew systems) |
To create a custom button setting using the above options, at most one
should be chosen from each of the 4 tables.
Recall the syntax for the MsgBox
command:
MsgBox(prompt[, buttons][, title][, helpfile, context])\xcode}
The value of buttons is given by the above four tables of values. There
are various ways in which these can be combined.
Suppose for example we wish to display the OK
and Cancel
buttons, with
the Critical Message
icon, where the second button is the default.
In this case the value of buttons in the MsgBox
command could be
\[1+16+256\quad\text{or}\quad 273\]
or
vbOKCancel + vbCritical + vbDefaultButton2
The latter is probably the most informative, but is also the
longest. We could chose to use a new variable to store the value; for
example if we defined an integer valued variable called DisplayOptions
then we could set
DisplayOptions = vbOKCancel + vbCritical + vbDefaultButton2
and then just use DisplayOptions
as the value for buttons in our
Message Box.
To illustrate the various icons available, as well as the different types of button references described above, we now give a series of examples. In Figure 13.1 we illustrate the Message Box obtained from the command
MsgBox("This is the Critical Message icon", 2+16, "Example 1")
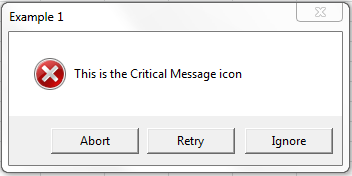
Figure 13.1: The Critical Message Icon
Figure 13.2 illustrates the result of using
MsgBox("This is the Warning Query icon", 37, "Example 2")
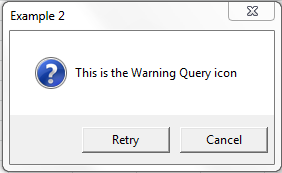
Figure 13.2: The Warning Query Icon
Figure 13.3 illustrates the result of using
MsgBox("This is the Warning Message icon", vbOKOnly + vbExclamation, "Example 3")
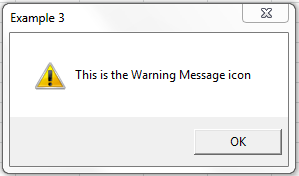
Figure 13.3: The Warning Message Icon
Figure illustrates the result of using
MsgBox("This is the Info Message icon", DispVar, "Example 4")
where DispVar = vbYesNo + vbInformation + vbDefaultButton1
.
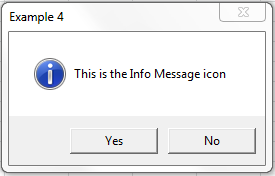
Figure 13.4: The Info Message Icon
If no icon value is chosen then no icon is displayed.
Recall from the lectures that the value of the variable assigned to
the MsgBox
statement depends on the button pressed by the user. This
is an integer valued variable; the possible values (and their names as
VBA constants) are given below.
Constant | Return Value | Selected button |
---|---|---|
vbOK |
1 | OK |
vbCancel |
2 | Cancel |
vbAbort |
3 | Abort |
vbRetry |
4 | Retry |
vbIgnore |
5 | Ignore |
vbYes |
6 | Yes |
`vbNo | 7 | No |
We end with a short example to illustrate the use of the return values.
Sub MsgBoxEx()
Dim DispVal As Integer
DispVal = vbCancel
Do While DispVal = vbCancel
DispVal = MsgBox("Would you like some tea?", vbYesNoCancel)
Loop
If DispVal = vbYes Then
DispVal = MsgBox("Tea not available!", 48)
Else
DispVal = MsgBox("Goodbye")
End If
End Sub
The above code gives a simple dialogue. It asks if the user wishes to have tea, and provides three buttons: Yes, No, and Cancel. Different messages are displayed depending on whether they choose Yes or No.
You should always make sure that your code can handle unexpected inputs from the user. For Message Boxes this is easy, as they can only press the buttons, but when we allow general inputs later this will be a more important consideration. Here we would naturally assume that the user will choose Yes or No, but the code is written so that if they choose Cancel then the Message Box will simply reappear.